Code to calculate Binomial Pricing for a period
- Richie Sawant
- Jan 10, 2021
- 4 min read
This MATLAB code calculates European and American Call and Put Option Price,
This is a really basic code that I wrote in like 2-3 hrs just wanted to share it!
Using Binomial Pricing we get a range of all the prices and we compare that to Black Scholes Price.
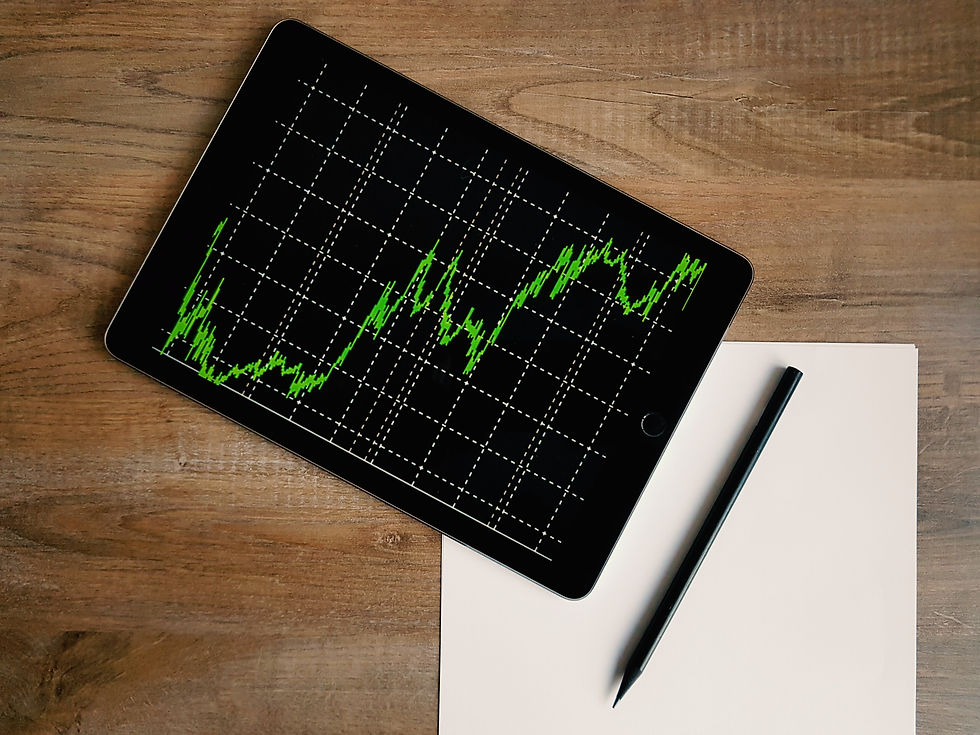
MATLAB Code:
cp = input( "1. European Call Option\n2. European Put Option\n3. American Call Option\n4. American Put Option\n Enter Your Option: ");
switch cp
case 1
y = input("No of years: ");
prompt1 = "Input the S0 value of the underlying stock: ";
S0 = input(prompt1);
prompt2 = "Input the K value of the option: ";
K = input(prompt2);
prompt3 = "Input the volatility of the underlying stock: ";
vol = input(prompt3);
prompt4 = "Input the risk free interest value: ";
r = input(prompt4);
prompt5 = "Input the number of steps: ";
n = input(prompt5);
clear prompt1 prompt2 prompt3 prompt4 prompt5
u = exp(vol * sqrt(y/n));
d = 1/u;
q = (exp((r*y)/n)-d)/(u-d);
p = 1-q;
F_Matrix = zeros(n,n);
%S_Matrix = zeros(n,n);
%SP_Matrix = zeros(n,n);
%L_Matrix = zeros(n,n);
%M_Matrix = ones(n,n);
for step = 0:n
%disp('Step No: ');
%step
for x = 0:(step)
%disp('No of values: ');
%x
S = S0*(u^(step-x))*(d^(x))*((1+r)^((step/n)*y));
S_Matrix(x+1, step+1) = S;
F_Matrix(x+1, step+1) = max(0,S-K*((1+r)^((-step/n)*y)));
SP_Matrix(x+1, step+1) = (nchoosek(step+1,(step-x+1)))*S*(q^(step-x+1))*(p^(x));
L_Matrix(x+1, step+1) = (nchoosek(step+1,(step-x+1)))*(max(0,S-K*((1+r)^((-step/n)*y))))*(q^(step-x+1))*(p^(x));
%M_Matrix(
%disp('Uppermost Value of Stock: ');
%UV
%disp('Lowest Value of Stock: ');
%LV
%disp('Uppermost Value of Option: ');
%max(0,UV-K)
%disp('Lowest Value of Option: ' );
%max(0,LV-K)
end
%S_Matrix = [S_Matrix; S_Matrix]
end
S_Matrix
%F_Matrix
%SP_Matrix
%L_Matrix
selected_step = input("Choose the step where you want to check the price of option: ");
Expected_Value = num2str(sum(L_Matrix(:,(selected_step+1))));
fprintf("The Price Value of the European Call Option at t=%s is: %s\n",num2str((selected_step/n)*y), Expected_Value)
[Call,Put] = blsprice(S0,K,r,(selected_step/n)*y,vol);
Call = num2str(Call);
fprintf("The Price Value of the European Call Option using BK at t=%s is: %s\n",num2str((selected_step/n)*y), Call)
%for x = 0:n
case 2
y = input("No of years: ");
prompt1 = "Input the S0 value of the underlying stock: ";
S0 = input(prompt1);
prompt2 = "Input the K value of the option: ";
K = input(prompt2);
prompt3 = "Input the volatility of the underlying stock: ";
vol = input(prompt3);
prompt4 = "Input the risk free interest value: ";
r = input(prompt4);
prompt5 = "Input the number of steps: ";
n = input(prompt5);
clear prompt1 prompt2 prompt3 prompt4 prompt5
u = exp(vol * sqrt(y/n));
d = 1/u;
q = (exp((r*y)/n)-d)/(u-d);
p = 1-q;
F_Matrix = zeros(n,n);
%S_Matrix = zeros(n,n);
%SP_Matrix = zeros(n,n);
%L_Matrix = zeros(n,n);
for step = 0:n
%disp('Step No: ');
%step
for x = 0:(step)
%disp('No of values: ');
%x
S = S0*(u^(step-x))*(d^(x))*((1+r)^((step/n)*y));
S_Matrix(x+1, step+1) = S;
F_Matrix(x+1, step+1) = max(0,K*((1+r)^((-step/n)*y))-S);
SP_Matrix(x+1, step+1) = (nchoosek(step+1,(step-x+1)))*S*(q^(step-x+1))*(p^(x));
L_Matrix(x+1, step+1) = (nchoosek(step+1,(step-x+1)))*(max(0,K*((1+r)^((-step/n)*y))-S))*(q^(step-x+1))*(p^(x));
%disp('Uppermost Value of Stock: ');
%UV
%disp('Lowest Value of Stock: ');
%LV
%disp('Uppermost Value of Option: ');
%max(0,UV-K)
%disp('Lowest Value of Option: ' );
%max(0,LV-K)
end
%S_Matrix = [S_Matrix; S_Matrix]
end
S_Matrix
%F_Matrix
%SP_Matrix
%L_Matrix
selected_step = input("Choose the step where you want to check the price of option: ");
Expected_Value = num2str(sum(L_Matrix(:,(selected_step+1))));
fprintf("The Price Value of the European Put Option at t=%s is: %s\n",num2str((selected_step/n)*y), Expected_Value)
[Call,Put] = blsprice(S0,K,r,(selected_step/n)*y,vol);
Put = num2str(Put);
fprintf("The Price Value of the European Put Option using BK at t=%s is: %s\n",num2str((selected_step/n)*y), Put)
case 3
y = input("No of years: ");
prompt1 = "Input the S0 value of the underlying stock: ";
S0 = input(prompt1);
prompt2 = "Input the K value of the option: ";
K = input(prompt2);
prompt3 = "Input the volatility of the underlying stock: ";
vol = input(prompt3);
prompt4 = "Input the risk free interest value: ";
r = input(prompt4);
prompt5 = "Input the number of steps: ";
n = input(prompt5);
clear prompt1 prompt2 prompt3 prompt4 prompt5
u = exp(vol * sqrt(y/n));
d = 1/u;
q = (exp((r*y)/n)-d)/(u-d);
p = 1-q;
F_Matrix = zeros(n,n);
S_Matrix = zeros(n,n);
SP_Matrix = zeros(n,n);
L_Matrix = zeros(n,n);
for step = 0:n
%disp('Step No: ');
%step
for x = 0:(step)
%disp('No of values: ');
%x
S = S0*(u^(step-x))*(d^(x))*((1+r)^((step/n)*y));
S_Matrix(x+1, step+1) = S;
F_Matrix(x+1, step+1) = max(0,S-K);
SP_Matrix(x+1, step+1) = (nchoosek(step+1,(step-x+1)))*S*(q^(step-x+1))*(p^(x));
L_Matrix(x+1, step+1) = (nchoosek(step+1,(step-x+1)))*(max(0,S-K))*(q^(step-x+1))*(p^(x));
%disp('Uppermost Value of Stock: ');
%UV
%disp('Lowest Value of Stock: ');
%LV
%disp('Uppermost Value of Option: ');
%max(0,UV-K)
%disp('Lowest Value of Option: ' );
%max(0,LV-K)
end
%S_Matrix = [S_Matrix; S_Matrix]
end
S_Matrix
F_Matrix
SP_Matrix
L_Matrix
selected_step = input("Choose the step where you want to check the price of option: ");
Expected_Value = num2str(sum(L_Matrix(:,(selected_step+1))));
fprintf("The Price Value of the American Call Option at t=%s is: %s\n",num2str((selected_step/n)*y), Expected_Value)
[Call,Put] = blsprice(S0,K,r,(selected_step/n)*y,vol);
Call = num2str(Call);
fprintf("The Price Value of the American Call Option using BK at t=%s is: %s\n",num2str((selected_step/n)*y), Call)
case 4
y = input("No of years: ");
prompt1 = "Input the S0 value of the underlying stock: ";
S0 = input(prompt1);
prompt2 = "Input the K value of the option: ";
K = input(prompt2);
prompt3 = "Input the volatility of the underlying stock: ";
vol = input(prompt3);
prompt4 = "Input the risk free interest value: ";
r = input(prompt4);
prompt5 = "Input the number of steps: ";
n = input(prompt5);
clear prompt1 prompt2 prompt3 prompt4 prompt5
u = exp(vol * sqrt(y/n));
d = 1/u;
q = (exp((r*y)/n)-d)/(u-d);
p = 1-q;
F_Matrix = zeros(n,n);
S_Matrix = zeros(n,n);
SP_Matrix = zeros(n,n);
L_Matrix = zeros(n,n);
for step = 0:n
%disp('Step No: ');
%step
for x = 0:(step)
%disp('No of values: ');
%x
S = S0*(u^(step-x))*(d^(x))*((1+r)^((step/n)*y));
S_Matrix(x+1, step+1) = S;
F_Matrix(x+1, step+1) = max(0,K-S);
SP_Matrix(x+1, step+1) = (nchoosek(step+1,(step-x+1)))*S*(q^(step-x+1))*(p^(x));
L_Matrix(x+1, step+1) = (nchoosek(step+1,(step-x+1)))*(max(0,K-S))*(q^(step-x+1))*(p^(x));
%disp('Uppermost Value of Stock: ');
%UV
%disp('Lowest Value of Stock: ');
%LV
%disp('Uppermost Value of Option: ');
%max(0,UV-K)
%disp('Lowest Value of Option: ' );
%max(0,LV-K)
end
%S_Matrix = [S_Matrix; S_Matrix]
end
S_Matrix
F_Matrix
SP_Matrix
L_Matrix
selected_step = input("Choose the step where you want to check the price of option: ");
Expected_Value = num2str(sum(L_Matrix(:,(selected_step+1))));
fprintf("The Price Value of the American Put Option at t=%s is: %s\n",num2str((selected_step/n)*y), Expected_Value)
[Call,Put] = blsprice(S0,K,r,(selected_step/n)*y,vol);
Put = num2str(Put);
fprintf("The Price Value of the American Put Option using BK at t=%s is: %s\n",num2str((selected_step/n)*y), Put)
otherwise
disp("Wrong Input, Please input 1,2,3 or 4")
end
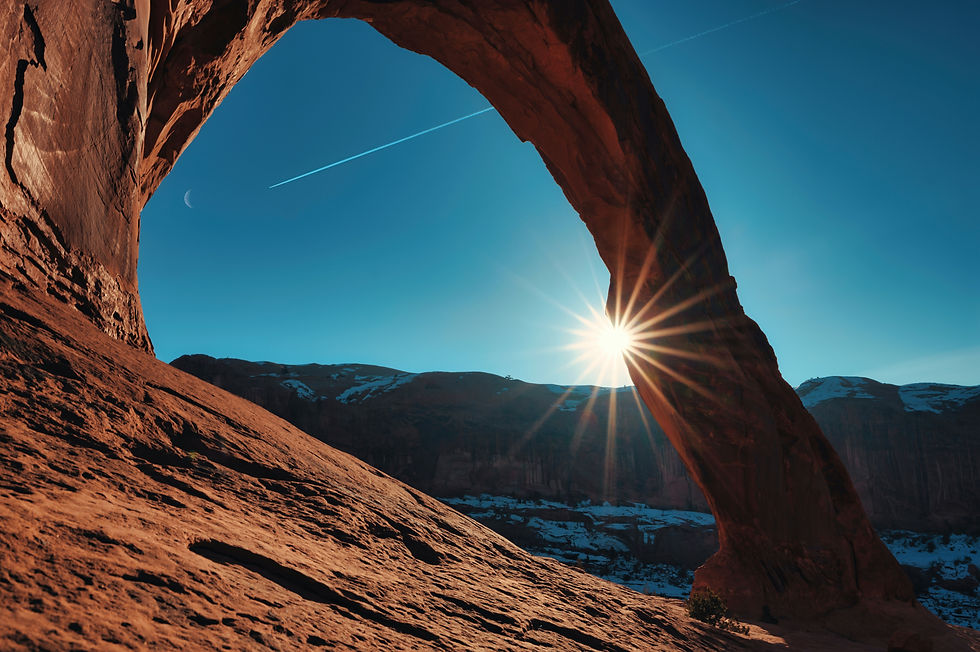
Happy New Year!!
Comments